3 minutes
Maya CMDS as .json file
I’m playing around with ways to make Tangle talk to Maya at the moment. I just implemented an RPC server to run in Maya, which works a bit easier than having to go throug a command port. So the next step I wanted to tackle was making nodes in Tangle that are basically maya.cmds commands. I started looking at maya.cmds stubs or something I could use to dynamically generate these nodes, but didn’t have much luck. Luckily, Maya’s python documentation is some of the best I’ve ever come across. It would be cool if I could grab data from there and use that generate Tangle nodes. So I spent the night writing a little scraper tool that scrapes the data on all the pages of http://help.autodesk.com/cloudhelp/2020/ENU/Maya-Tech-Docs/CommandsPython/index.html and dumps them into a .json file.
This is how the .json is structured:
{
"polyCube": {
"url": "http://help.autodesk.com/cloudhelp/2020/ENU/Maya-Tech-Docs/CommandsPython/polyCube.html",
"undoable": true,
"queryable": true,
"editable": true,
"arguments": [
{
"name": "axis",
"type": "[linear, linear, linear]",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "caching",
"type": "boolean",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "constructionHistory",
"type": "boolean",
"in_create_mode": true,
"in_query_mode": true
},
{
"name": "createUVs",
"type": "int",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "depth",
"type": "linear",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "height",
"type": "linear",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "name",
"type": "string",
"in_create_mode": true
},
{
"name": "nodeState",
"type": "int",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "object",
"type": "boolean",
"in_create_mode": true
},
{
"name": "subdivisionsDepth",
"type": "int",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "subdivisionsHeight",
"type": "int",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "subdivisionsWidth",
"type": "int",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "subdivisionsX",
"type": "int",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "subdivisionsY",
"type": "int",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "subdivisionsZ",
"type": "int",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "texture",
"type": "int",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
},
{
"name": "width",
"type": "linear",
"in_create_mode": true,
"in_query_mode": true,
"in_edit_mode": true
}
],
"example": "import maya.cmds as cmds\n\ncmds.polyCube( sx=10, sy=15, sz=5, h=20 )\n#result is a 20 units height rectangular box\n#with 10 subdivisions along X, 15 along Y and 20 along Z.\n\ncmds.polyCube( sx=5, sy=5, sz=5 )\n#result has 5 subdivisions along all directions, default size\n\n# query the width of a cube\nw = cmds.polyCube( 'polyCube1', q=True, w=True )\n\n ",
"description": "The cube command creates a new polygonal cube.",
"return_type": "string[]",
"return_doc": "Object name and node name."
}
}
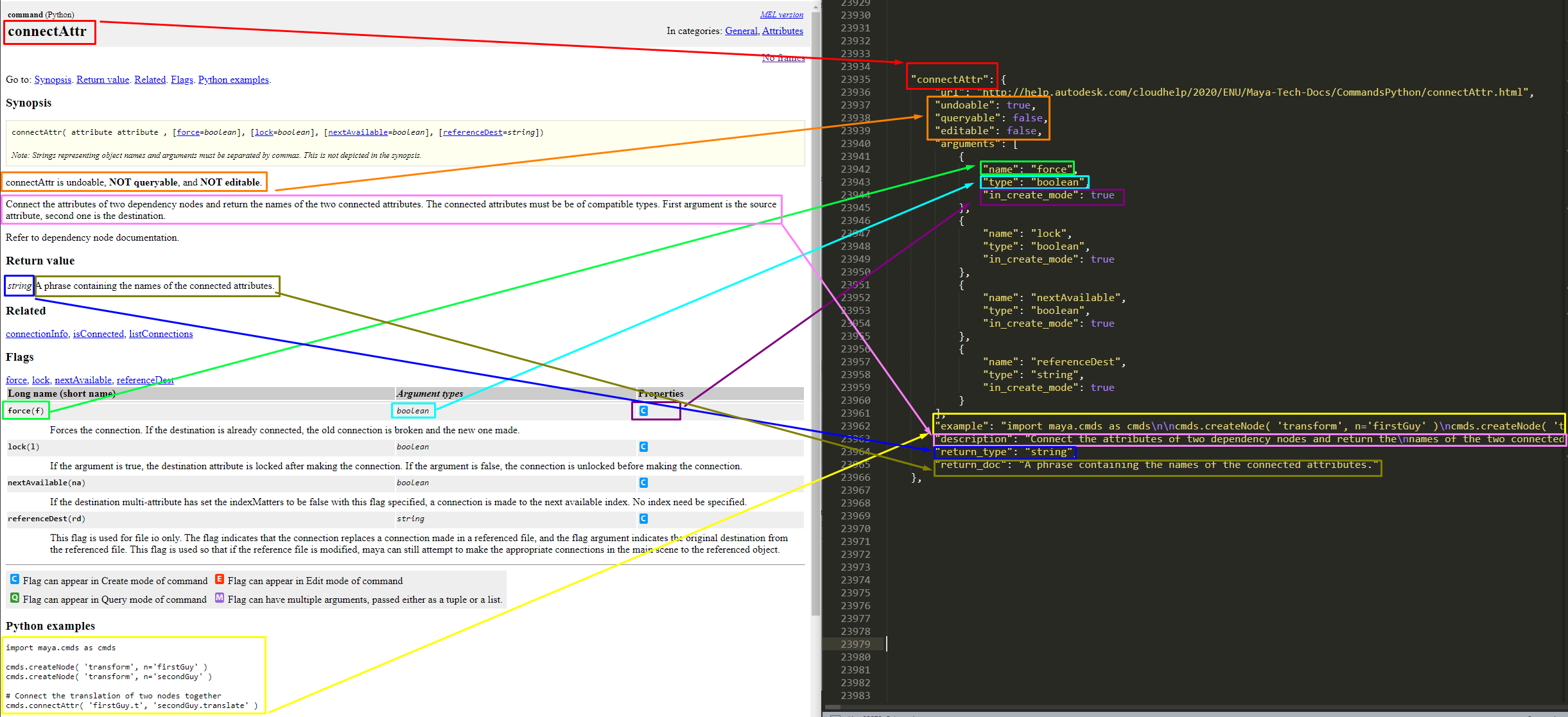
Feel free to grab it if you think you have any use for it: commands.json